PShell - A Runtime Environment for Sharp Pocket Computers 
What's that ?
PShell is a runtime environment for Sharp pocket computers. For the user,
it allows to run machine language programs in a very comfortable way.
For the developer, it makes it more easy the write machine language
programs which are easy portable from one model to another.
PShell itself is portable to all Sharp pocket computers with the SC61860 CPU
and at least 8 kByte of RAM, e.g. PC-1262, PC-1402 and PC-1350 (with memory
card). I started to implement it for the PC-1350 and the PC-1262, because
there are very useful emulators available.
What problems does it address?
On sharp pocket computers, it is very hard to handle machine language programs.
You need to know memory addresses, move around basic pointers and it is not
possible to have two machine language programs in memory if they were built for
the same memory region. Also you need to know various PEEK, POKE and CALL
addresses. It is very hard to delete programs from memory or add some more.
For the developer, it is very hard to write portable code, because there is
no common way of accessing keyboard, display, sio, etc. BASIC programs
must be modified if a machine language library changes its CALL addresses or
is ported to another PC-model.
How does PShell solve this Problems?
For the User:
Once installed, you need to remember only one single CALL address. This address is displayed
during the setup process, so write it into your hard cover :-). This CALL leads you to a
menu containing all installed PShell programs. Just select one with the cursor-up and cursor-down
keys and press enter. Simple, isn't it?
|
|
For the BASIC Programmer:
If you have a machine language library in PShell style, you can call functions by name with
a standard interface (not by address, as usual). You need to know only the base address from
the PShell installation (same as CALL address for users). Here is an example how to call a PShell
program/library function:
690 X=8244:REM PSHELL CALL ADDR
...
26000 PAUSE "EXECUTE FDISK .."
26005 S$="FDISK":CALL X+12:CALL X+8
This example is taken from the PShell setup program (which calls this program to organize the
memory). The exact meaning of the call interface is described later.
For the Machine Language Developer:
PShell has a powerful API with many hardware related functions. They are similar on all models. E.g.
CALL ps_putchar
writes a character to the display. You don't need to know the position of the character table,
addresses of the inner ROM, etc. You can write very portable code with conditional assembly,
because there are very much built-in defines. Example:
app315:
.if __PC_HAS_LOWERCASE_CHARS__
.ascii "Sound is "
.db 0x00
.ascii "on"
.db 0x00
.ascii "off"
.db 0x00
.else
.ascii "SOUND IS "
.db 0x00
.ascii "ON"
.db 0x00
.ascii "OFF"
.db 0x00
.endif
This is a code snippet from the sound control program (some strings to display when switching sound
on and off). __PC_HAS_LOWERCASE_CHARS__ is set when the pocket computer supports lower case letters.
When assembled for another model which doesn't support lower case letters, all strings will be upper
case.
It is easy to modify the representation of the program in the PShell main menu (program name and icon).
PShell programs must have a special header to specify this. See the 'Hello World' program later on.
Beside all these advantages, there is also a drawback which should be mentioned: PShell programs
must be fully relocatable. This means, they may not contain any jumps or calls to absolute addresses
(except PShell functions and other memory locations pointed to by PShell defines).
This sounds hard to do, but PShell has some supporting functions (getting the address of the own program,
relative to absolute address calculation ...). Also see the 'Hello World' program to get a sample for this.
You don't need the full PShell sources to assemble a PShell program. There is a header file which must
be includes. PShell programs are not binary portable, that means you must reassemble the program with
a different header fill if you want to use it for another model (or the same model with a different
memory card).
PShell programs may be packaged with a basic loader which gets the right memory location automatically
from the PShell installation (also the available space is checked). Maybe I (or someone other who finds
the motivation to do this) write a sio loader program which can read IHX files direct in the future,
because basic loaders are rather slow, especially if you transfer them over the tape interface.
You need the as61860 assembler to build programs for PShell.
Where did you get the idea from to do this?
From TiCalc. There is a similar project for the TI-85 calculator called ZShell.
Which license applies to PShell?
It comes with GPL and without warranty of any kind.
HOWTO install it?
Browse the File Archive and look for PSHELL.BAS in the folder matching to
your model. If you can't find it, it is most likely because I did not port it by now, this time I
only assembled the PC-1350 version with 8 kByte memory extension.
Transfer it to your PC or type it in (wonder if there is really someone doing this ...) and execute
it. Just follow the instructions on the display now, then wait ... wait ... wait ...
Hints:
-
The setup program was designed to be most portable, so don't tell me it look ugly ...
-
You can install PShell without check of data integrity by typing "NOCHK" and DEF-S.
This is faster, but more insecure.
Where are all the PShell Programs?
They are waiting to be developed by YOU :-)
These are waiting for being implemented:
-
RS232 PShell program loader/saver (IHX files)
-
RS232 Basic program loader/saver (11-pin interface, for models without 15-pin interface
or for persons who want to use a 11-pin level converter on a model with 15-pin interface)
-
Some machine language games like 'Airplane'
-
Petools port for SPhell (not only for PC-1403(H) then)
-
Help function like on PC-1262
-
Audio record/playback (Yes, this is really possible!)
-
A program to identify Austrian and German car license plates
-
Record level meter application (VU meter)
-
Greek letters application (like on PC-E220)
-
RS-232 terminal program (hmm, hard to do)
These are already included into the standard package:
-
Sound on/off
-
Shutdown
-
PShell program delete
-
Renew/reclear (Renew work and has improvement potential, Reclear not implemented)
-
Memory manager (FDISK)
Which Machine Language Functions are available for PShell Programs?
PShell provides the following functionality for machine language programs:
-
Keyboard: Raw read, blocking, non blocking or single matrix line read for e.g. fast games (character read maybe in the future).
-
Display: Character/string write, graphics output (like GPRINT), including LC display address calculation from cursor position and BitBlt function.
-
RS232: Character/string read and write on both 11-pin and 15-pin interface.
-
Beeper: Short dual tone beep, beeps with variable frequency.
-
Power management (APM :-) ): Soft power off, power saving in keyboard loops.
-
Runtime: Relative to absolute address calculation (to make programs full relocatable).
-
Conversion: Unsigned int to string conversion and vice versa.
-
String manipulation: String compare/startswith function.
-
Error handling: PShell programs may throw ERROR n messages up to the BASIC prompt.
Appendix
BASIC CALL Interface
XXXX is the base address as shown during setup.
CALL/PEEK/POKE
|
Description
|
POKE XXXX-4, PEEK XXXX-4
|
call interface parameter (byte or word, read and write)
|
CALL XXXX
|
normal call (main menue)
|
CALL XXXX+4
|
remembers the parameter of the
WAIT command (for later restore)
|
CALL XXXX+8
|
calls the program looked up with
CALL XXXX+12. A parameter may be given with the
WAIT command (the WAIT command is restored to
the value stored with CALL XXXX+4 after this)
|
CALL XXXX+12
|
lookup a program with the name
given in S$ and perform CALL XXXX+4 inplicit
(throws an ERROR 9 if not found)
|
CALL XXXX+16
|
returns next free address for
pshell programs in std. var. S$ 1)
|
CALL XXXX+20
|
CALL XXXX+20 returns the start of the BASIC
RAM in std. var. S$ 1)
|
Hello World Program
; --8x------------------------------------------------
; Start of seventh application - Hello World
; ----------------------------------------------------
app7st:
.dw app7end-app7st ; size of the programm
.if __PC_HAS_LOWERCASE_CHARS__
.ascii "HelloW" ; name of the programm
.else
.ascii "HELLOW" ; name of the programm
.endif
.db 0x00 ; end of string
.db 0x05 ; number of graphic
; icon bytes
.dw 0x1455 ; icon pattern
.dw 0x5555
.db 0x14
CALL ps_clrscr
LIA <(app701-app7st)
LIB >(app701-app7st)
CALL ps_rel_ab2abs_x
CALL ps_putstr
LIA 0xff
CALL ps_sleep
RTN
app701:
.if __PC_HAS_LOWERCASE_CHARS__
.ascii "Hello World!"
.db 0x00
.else
.ascii "HELLO WORLD!"
.db 0x00
.endif
app7end:
; .dw 0x0000 ; trailing 0 to indicate
; the end of the pshell
; program series; this
; may be overwritten by
; the next program in
; memory
Included Programs
All programs are accessible through the main menu you can see on the right side.
|
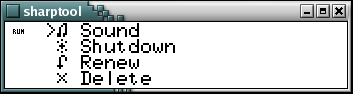
|
Sound
|
With the Sound program you can turn the beeper on and off again.
Note: this works only for programs using PSHELL.
|
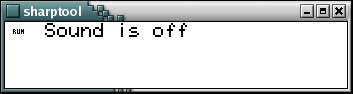
|
Delete
|
This program deletes the last program listed in the menu. To change
the order in the menu you have to delete and re-install the programs
in the order you want. If you delete the Delete program
itself, you can not delete the remaining programs in the menu.
|
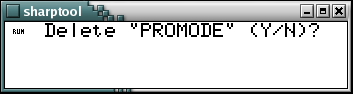
|
FDISK
|
This program reserves memory for PSHELL programs.
|
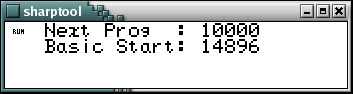
|
Screenshots
Screenshots from Setup Program
Screenshot running on the PC-1262 Emulation